Installation
Add the package to your project using Flutter pub:
Installation
flutter pub add flutwid_ui
Slider
Installation
dart run flutwid_ui:flutwid_ui add slide_button
Usage
// Example usage
class SlideButtonExample extends StatelessWidget {
const SlideButtonExample({super.key});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(20.0),
child: SlideButton(
onConfirm: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('Action confirmed!')),
);
},
),
);
}
}
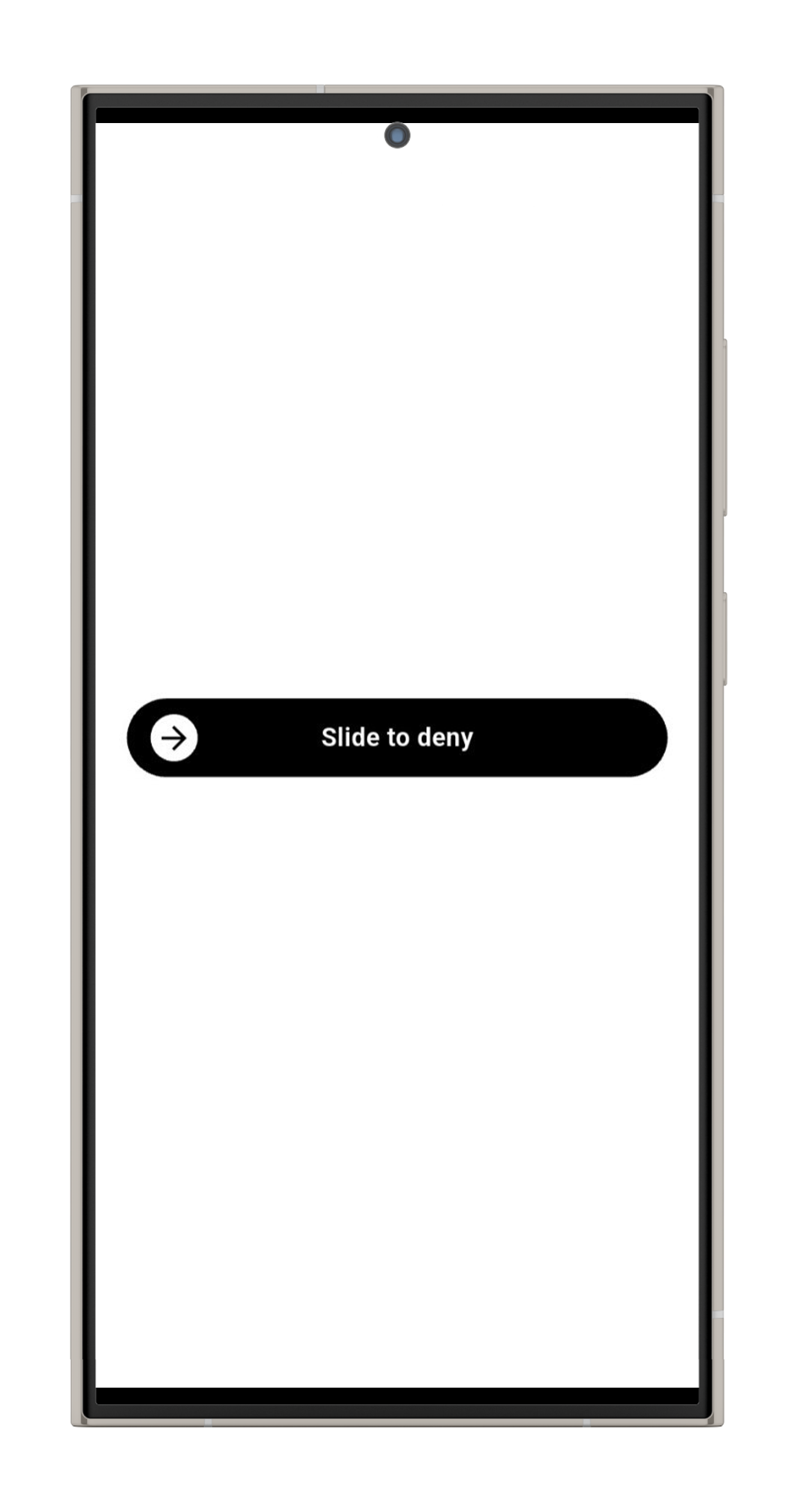
Time Picker
Installation
dart run flutwid_ui:flutwid_ui add time_picker
Usage
TimePicker(
onTimeChanged: (selectedTime) {
print('Selected time: ' + selectedTime.hour + ':' + selectedTime.minute);
},
)
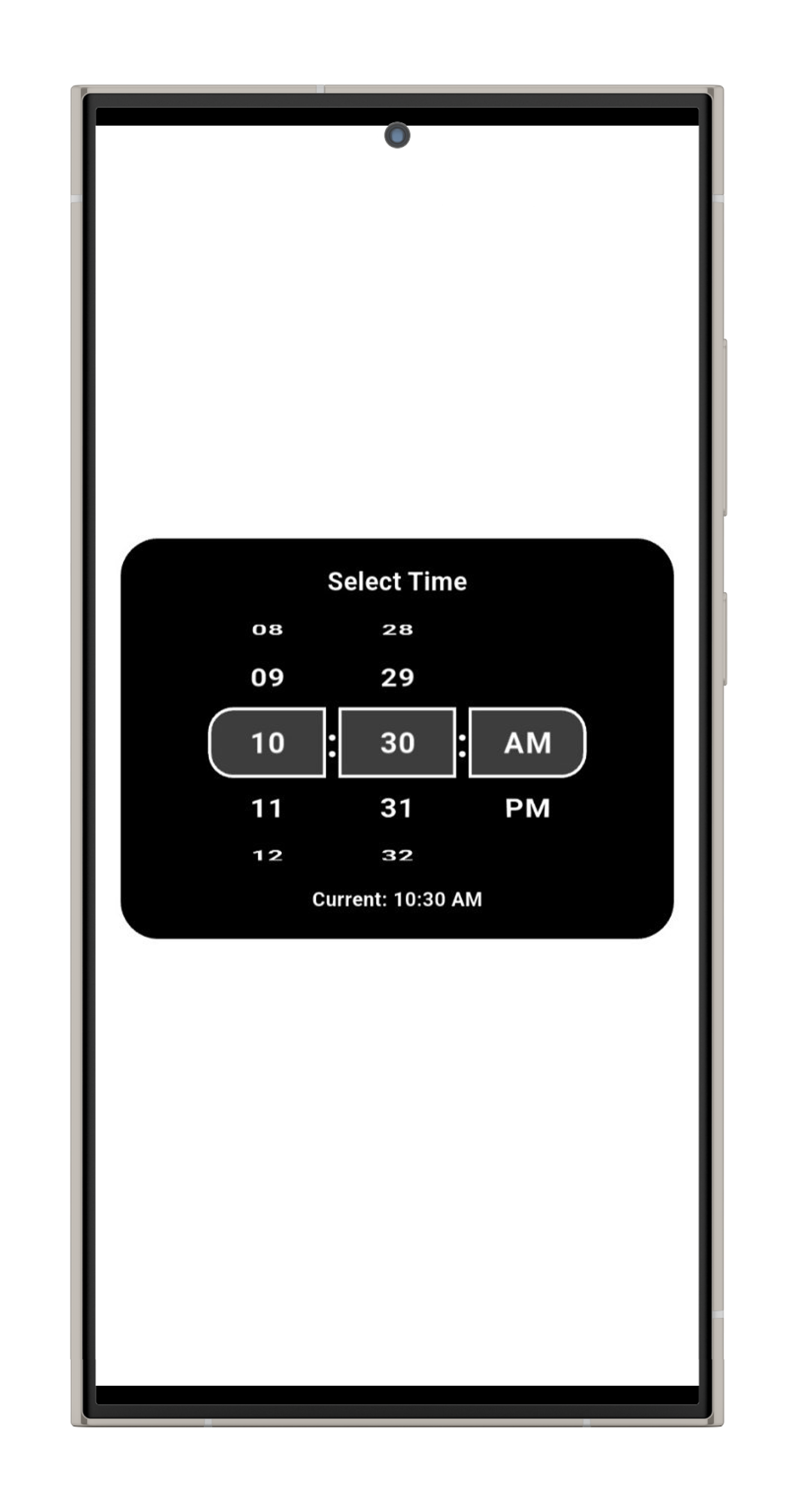
Password Field
Installation
dart run flutwid_ui:flutwid_ui add password_field
Usage
// Password Field
PasswordField(
hintText: 'Enter your password',
labelText: 'Password',
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please enter your password';
}
return null;
},
)
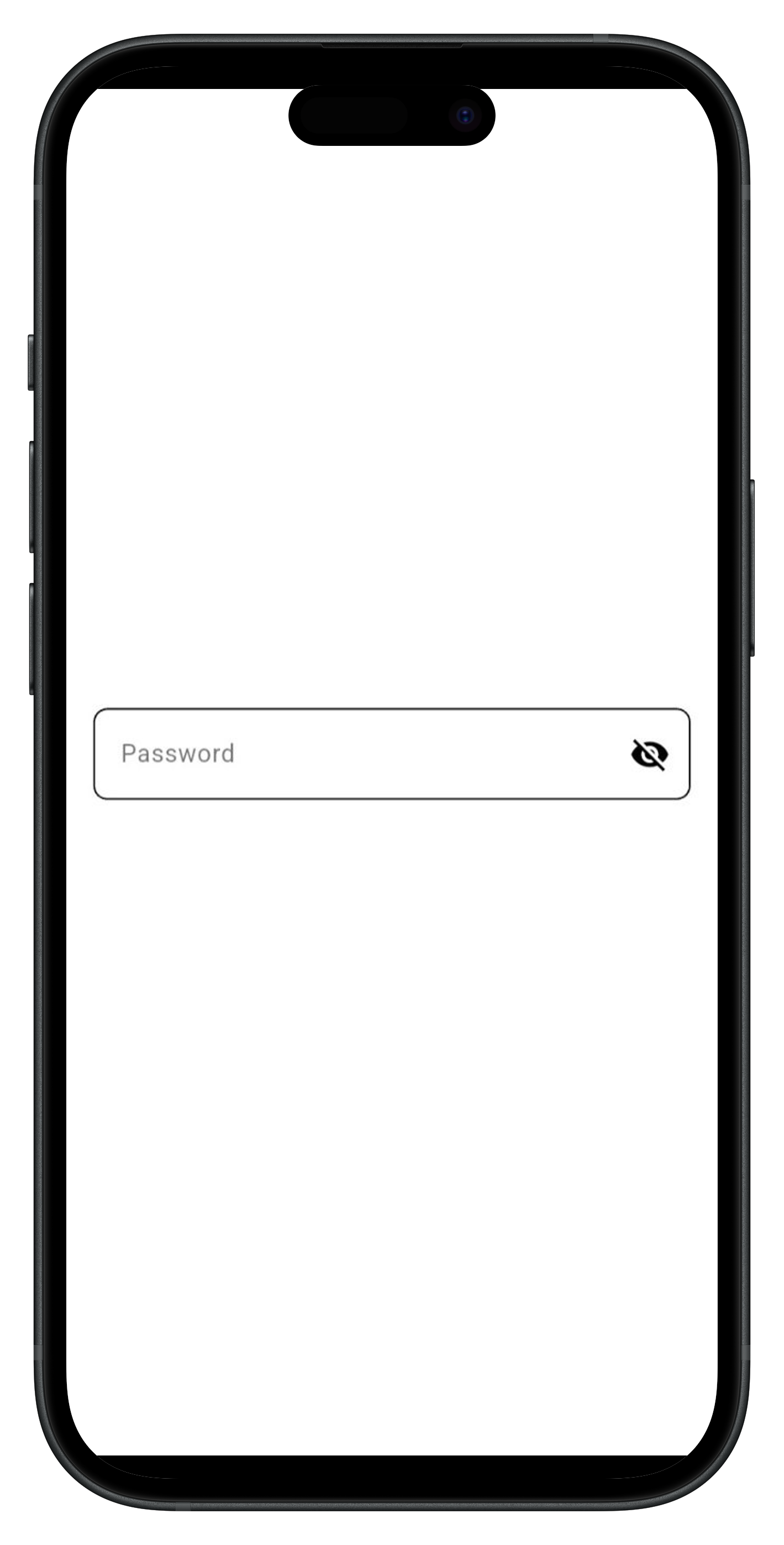
Sign In Form
Installation
dart run flutwid_ui:flutwid_ui add signin_form
Usage
SigninForm(
onSignInPressed: (email, password) {
// Handle sign in logic
print('Email: ' + email + ', Password: ' + password);
},
onForgotPasswordPressed: () {
// Handle forgot password logic
print('Forgot password pressed');
},
)
// Customized usage
SigninForm(
backgroundColor: Colors.black,
primaryColor: Colors.white,
buttonColor: Colors.white,
buttonTextColor: Colors.black,
borderRadius: 12.0,
horizontalPadding: 24.0,
emailHintText: 'Enter your email',
passwordHintText: 'Enter your password',
signInButtonText: 'Login',
onSignInPressed: (email, password) {
// Handle sign in logic
},
)
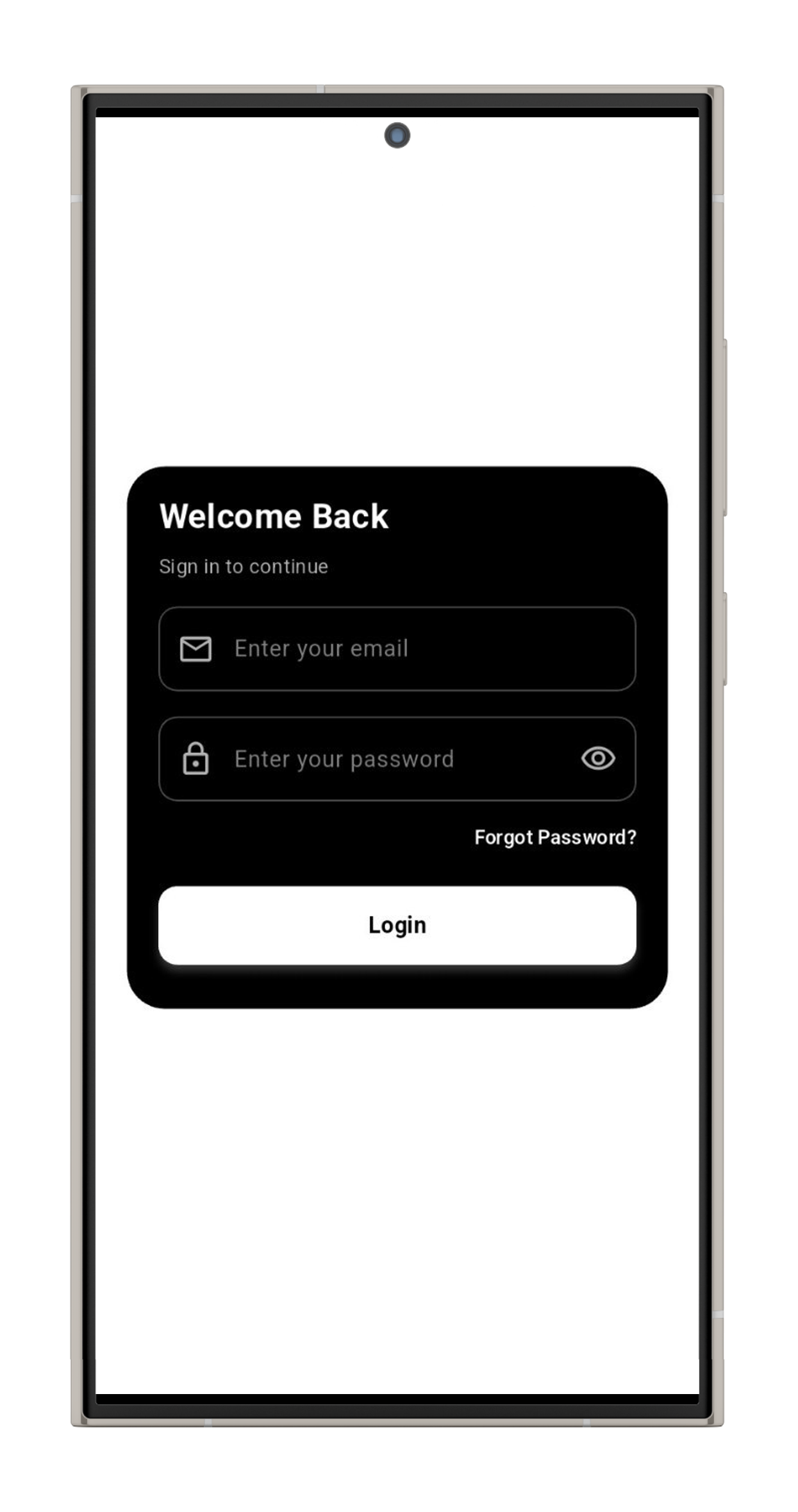
Toast
Installation
dart run flutwid_ui:flutwid_ui add toast_notification
Usage
ElevatedButton(
onPressed: () {
// Show a simple toast when button is clicked
ToastNotification.show(
context,
message: 'This is a simple toast message',
);
},
child: const Text('Show Toast'),
)
// Success toast at the top
ToastNotification.show(
context,
message: 'Success! Your action was completed',
icon: Icons.check_circle,
iconColor: Colors.green,
position: ToastPosition.top,
);
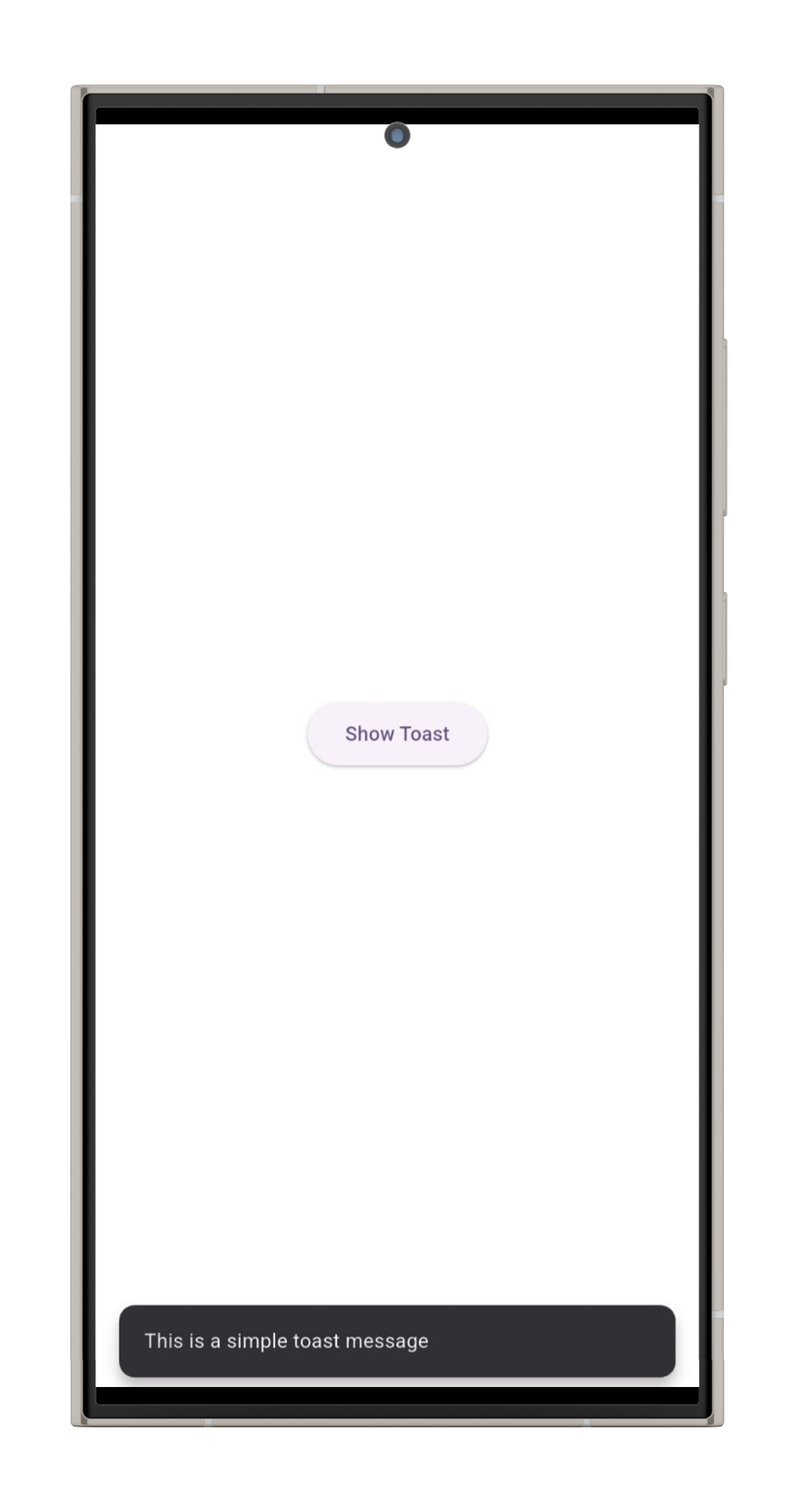